Badge
Badge is a small overlapped UI item which indicates a status, notification, or event that appears in relativity with the underlying object.
import { Badge } from "rizzui";
Default
The default style of Badge component.
import { Badge } from "rizzui";
export default function App() {
return <Badge>Badge</Badge>;
}
Variants
You can change the style of the Badge using variant property.
import { Badge } from "rizzui";
export default function App() {
return (
<>
<Badge variant="outline">Badge</Badge>
<Badge variant="flat">Badge</Badge>
<Badge>Badge</Badge>
</>
);
}
Sizes
You can change the size of the Badge using size property.
import { Badge } from "rizzui";
export default function App() {
return (
<>
<Badge size="sm">Badge</Badge>
<Badge>Badge</Badge>
<Badge size="lg">Badge</Badge>
<Badge size="xl">Badge</Badge>
</>
);
}
Rounded
You can change the border radius of the Badge using rounded property.
import { Badge } from "rizzui";
export default function App() {
return (
<>
<Badge rounded="none">Badge</Badge>
<Badge rounded="sm">Badge</Badge>
<Badge rounded="md">Badge</Badge>
<Badge rounded="lg">Badge</Badge>
<Badge>Badge</Badge>
</>
);
}
Colors
You can change the color of the Badge using color property.
Badge
Badge
Badge
Badge
Badge
Badge
Badge
Badge
Badge
Badge
import { Badge } from "rizzui";
export default function App() {
return (
<>
{/* variant solid / default */}
<div className="flex items-center justify-around gap-3 w-full flex-wrap mb-10">
<Badge>Badge</Badge>
<Badge color="secondary">Badge</Badge>
<Badge color="info">Badge</Badge>
<Badge color="warning">Badge</Badge>
<Badge color="danger">Badge</Badge>
<Badge color="success">Badge</Badge>
</div>
{/* variant flat */}
<div className="flex items-center justify-around gap-3 w-full flex-wrap mb-10">
<Badge variant="flat">Badge</Badge>
<Badge
variant="flat"
color="secondary"
>
Badge
</Badge>
<Badge
variant="flat"
color="info"
>
Badge
</Badge>
<Badge
variant="flat"
color="warning"
>
Badge
</Badge>
<Badge
variant="flat"
color="danger"
>
Badge
</Badge>
<Badge
variant="flat"
color="success"
>
Badge
</Badge>
</div>
{/* variant outline */}
<div className="flex items-center justify-around gap-3 w-full flex-wrap">
<Badge variant="outline">Badge</Badge>
<Badge
variant="outline"
color="secondary"
>
Badge
</Badge>
<Badge
variant="outline"
color="info"
>
Badge
</Badge>
<Badge
variant="outline"
color="warning"
>
Badge
</Badge>
<Badge
variant="outline"
color="danger"
>
Badge
</Badge>
<Badge
variant="outline"
color="success"
>
Badge
</Badge>
</div>
</>
);
}
Dot
You can render Badge as dot using renderAsDot property.
Primary
Secondary
Danger
Warning
Success
Info
import { Badge, Text } from "rizzui";
export default function App() {
return (
<>
<div className="flex items-center gap-2">
<Badge renderAsDot />
<Text>Primary</Text>
</div>
<div className="flex items-center gap-2">
<Badge
renderAsDot
color="secondary"
/>
<Text>Secondary</Text>
</div>
<div className="flex items-center gap-2">
<Badge
renderAsDot
color="danger"
/>
<Text>Danger</Text>
</div>
<div className="flex items-center gap-2">
<Badge
renderAsDot
color="warning"
/>
<Text>Warning</Text>
</div>
<div className="flex items-center gap-2">
<Badge
renderAsDot
color="success"
/>
<Text>Success</Text>
</div>
<div className="flex items-center gap-2">
<Badge
renderAsDot
color="info"
/>
<Text>Info</Text>
</div>
</>
);
}
With Icon
You can use Badge to make a ecommerce cart counter component.
99
99+
999+
import { Badge } from "rizzui";
import { ShoppingBagIcon, ShoppingCartIcon } from "@heroicons/react/24/outline";
export default function App() {
return (
<>
<div className="relative inline-flex">
<ShoppingBagIcon
className="h-auto w-8"
strokeWidth={1.2}
/>
<Badge
size="sm"
enableOutlineRing
className="absolute right-0 top-0 -translate-y-1/3 translate-x-1/2"
>
99
</Badge>
</div>
<div className="relative ml-3 inline-flex">
<ShoppingCartIcon
className="h-auto w-8"
strokeWidth={1.2}
/>
<Badge
size="sm"
color="success"
enableOutlineRing
className="absolute right-0 top-0 -translate-y-1/3 translate-x-1/2"
>
99+
</Badge>
</div>
<div className="relative ml-3 inline-flex">
<ShoppingBagIcon
className="h-auto w-8"
strokeWidth={1.2}
/>
<Badge
size="sm"
color="danger"
enableOutlineRing
className="absolute right-0 top-0 -translate-y-1/3 translate-x-1/2"
>
999+
</Badge>
</div>
</>
);
}
With Avatar
You can use the Badge with Avatar component to show the current state of user.
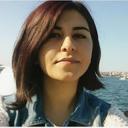

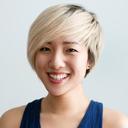
import { Badge, Avatar } from "rizzui";
export default function App() {
return (
<>
<div className="relative inline-flex">
<Avatar
name="John Doe"
size="lg"
src="https://randomuser.me/api/portraits/women/40.jpg"
/>
<Badge
size="lg"
renderAsDot
color="success"
enableOutlineRing
className="absolute right-0 top-0 -translate-y-[25%]"
/>
</div>
<div className="relative ml-3 inline-flex">
<Avatar
name="John Doe"
size="lg"
src="https://randomuser.me/api/portraits/women/43.jpg"
/>
<Badge
size="lg"
renderAsDot
color="danger"
enableOutlineRing
className="absolute right-0 bottom-0 -translate-y-[25%]"
/>
</div>
<div className="relative ml-3 inline-flex">
<Avatar
name="John Doe"
size="lg"
src="https://randomuser.me/api/portraits/women/44.jpg"
/>
<Badge
size="lg"
renderAsDot
color="warning"
enableOutlineRing
className="absolute right-0 top-0 -translate-y-[30%]"
/>
</div>
</>
);
}
API Reference
Badge Props
Here is the API documentation of the Badge component. You can use props like id, title, onClick etc.
Props | Type | Description | Default |
---|---|---|---|
children | React.ReactNode | Accepts everything React can render | __ |
variant | BadgeVariants | Set the Badge variants | "solid" |
size | BadgeSizes | Set the size of the component. "sm" is equivalent to the dense Badge styling. | "md" |
rounded | BadgeRounded | Set border radius of the Badge component | "full" |
color | BadgeColors | Change Badge color | "primary" |
enableOutlineRing | boolean | Set a outline ring. It is useful for the overlapping UI. | __ |
renderAsDot | boolean | Render badge as a dot | __ |
className | string | Add custom classes for extra style | __ |
Badge Variants
type BadgeVariants = "solid" | "flat" | "outline";
Badge Sizes
type BadgeSizes = "sm" | "md" | "lg" | "xl";
Badge Rounded
type BadgeRounded = "none" | "sm" | "md" | "lg" | "full";
Badge Colors
type BadgeColors = "primary" | "secondary" | "danger" | "info" | "success" | "warning";