Avatar
Avatar is a small UI item which represents user profile picture, or event that appears in relativity with the underlying object.
import { Avatar } from "rizzui";
Default
The default style of the Avatar.
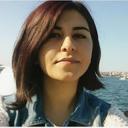
import { Avatar } from "rizzui";
export default function App() {
return (
<Avatar
name="Jane Doe"
src="https://randomuser.me/api/portraits/women/40.jpg"
/>
);
}
Variants
You can add text or avatar icon to Avatar component using initials or src property.
JD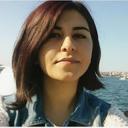
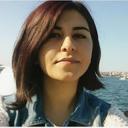
import { Avatar } from "rizzui";
export default function App() {
return (
<>
<Avatar
name="John Doe"
initials="AB"
/>
<Avatar
src="https://randomuser.me/api/portraits/women/40.jpg"
name="John Doe"
/>
</>
);
}
Sizes
You can change the size of the Avatar using size property.
SM
L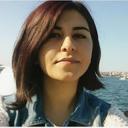

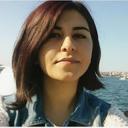
import { Avatar } from "rizzui";
export default function App() {
return (
<>
<Avatar
name="John Doe"
initials="SM"
size="sm"
/>
<Avatar
src="https://randomuser.me/api/portraits/men/40.jpg"
name="John Doe"
/>
<Avatar
name="John Doe"
initials="L"
size="lg"
/>
<Avatar
name="John Doe"
src="https://randomuser.me/api/portraits/women/40.jpg"
size="xl"
/>
</>
);
}
Rounded
You can change the border radius of the Avatar using rounded property.
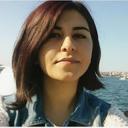



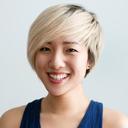
import { Avatar } from "rizzui";
export default function App() {
return (
<>
<Avatar
src="https://randomuser.me/api/portraits/women/40.jpg"
name="John Doe"
rounded="none"
/>
<Avatar
name="John Doe"
src="https://randomuser.me/api/portraits/women/41.jpg"
rounded="sm"
/>
<Avatar
rounded="md"
src="https://randomuser.me/api/portraits/women/42.jpg"
name="John Doe"
/>
<Avatar
rounded="lg"
src="https://randomuser.me/api/portraits/women/43.jpg"
name="John Doe"
/>
<Avatar
src="https://randomuser.me/api/portraits/women/44.jpg"
name="John Doe"
/>
</>
);
}
Colors
You can change the color of the Avatar using color property.
SMSMSMSMSMSMSM
import { Avatar } from "rizzui";
export default function App() {
return (
<>
<Avatar
name="John Doe"
initials="SM"
/>
<Avatar
name="John Doe"
initials="SM"
color="primary"
/>
<Avatar
name="John Doe"
initials="SM"
color="secondary"
/>
<Avatar
name="John Doe"
initials="SM"
color="danger"
/>
<Avatar
name="John Doe"
initials="SM"
color="warning"
/>
<Avatar
name="John Doe"
initials="SM"
color="success"
/>
<Avatar
name="John Doe"
initials="SM"
color="info"
/>
</>
);
}
Borders
You can add border to Avatar by adding extra className using className property.



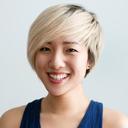
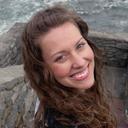
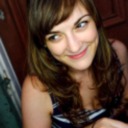
import { Avatar } from "rizzui";
export default function App() {
return (
<>
<Avatar
src="https://randomuser.me/api/portraits/women/41.jpg"
name="John Doe"
className="ring-2 ring-primary ring-offset-background ring-offset-2"
/>
<Avatar
src="https://randomuser.me/api/portraits/women/42.jpg"
name="John Doe"
className="ring-2 ring-secondary ring-offset-background ring-offset-2"
/>
<Avatar
src="https://randomuser.me/api/portraits/women/43.jpg"
name="John Doe"
className="ring-2 ring-red ring-offset-background ring-offset-2"
/>
<Avatar
src="https://randomuser.me/api/portraits/women/44.jpg"
name="John Doe"
className="ring-2 ring-orange ring-offset-background ring-offset-2"
/>
<Avatar
src="https://randomuser.me/api/portraits/women/45.jpg"
name="John Doe"
className="ring-2 ring-green ring-offset-background ring-offset-2"
/>
<Avatar
src="https://randomuser.me/api/portraits/women/46.jpg"
name="John Doe"
className="ring-2 ring-blue ring-offset-background ring-offset-2"
/>
</>
);
}
Online Status
You can use Badge with Avatar component for online status.
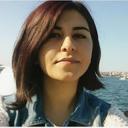

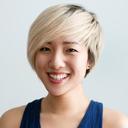
import { Avatar, Badge } from "rizzui";
export default function App() {
return (
<>
<div className="relative inline-flex">
<Avatar
size="lg"
name="John Doe"
src="https://randomuser.me/api/portraits/women/40.jpg"
/>
<Badge
renderAsDot
color="success"
enableOutlineRing
size="lg"
className="absolute right-0 top-0 -translate-y-[25%]"
/>
</div>
<div className="relative ml-3 inline-flex">
<Avatar
size="lg"
name="John Doe"
src="https://randomuser.me/api/portraits/women/43.jpg"
/>
<Badge
renderAsDot
color="danger"
enableOutlineRing
size="lg"
className="absolute right-0 top-0 -translate-y-[25%]"
/>
</div>
<div className="relative ml-3 inline-flex">
<Avatar
size="lg"
name="John Doe"
src="https://randomuser.me/api/portraits/women/44.jpg"
/>
<Badge
renderAsDot
color="warning"
enableOutlineRing
size="lg"
className="absolute right-0 top-0 -translate-y-[30%]"
/>
</div>
</>
);
}
Group
You can use Avatar component to create a group of avatars.
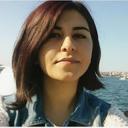



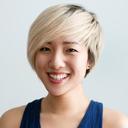
+5
import { Avatar } from "rizzui";
export default function App() {
return (
<>
<Avatar
customSize="42"
name="John Doe"
src="https://randomuser.me/api/portraits/women/40.jpg"
className="relative inline-flex object-cover"
/>
<Avatar
customSize="42"
name="John Doe"
src="https://randomuser.me/api/portraits/women/41.jpg"
className="relative inline-flex -translate-x-[5px] object-cover ring-2 ring-background"
/>
<Avatar
customSize="42"
name="John Doe"
src="https://randomuser.me/api/portraits/women/42.jpg"
className="relative inline-flex -translate-x-[10px] object-cover ring-2 ring-background"
/>
<Avatar
customSize="42"
name="John Doe"
src="https://randomuser.me/api/portraits/women/43.jpg"
className="relative inline-flex -translate-x-[15px] object-cover ring-2 ring-background"
/>
<Avatar
customSize="42"
name="John Doe"
src="https://randomuser.me/api/portraits/women/44.jpg"
className="relative inline-flex -translate-x-[20px] object-cover ring-2 ring-background"
/>
<div className="bordered relative inline-flex h-[42px] w-[42px] -translate-x-[25px] items-center justify-center rounded-full object-cover text-sm font-medium text-gray-900">
+5
</div>
</>
);
}
API Reference
Avatar Props
Here is the API documentation of the Avatar component.
Props | Type | Description | Default |
---|---|---|---|
name | string | Will be used to generate avatar initials based on the person name when image is missing | __ |
initials | string | Display custom text when image is missing | __ |
src | string | Image soruce (local or remote) | __ |
color | AvatarColors | Modify avatar color; by default, avatars have randomly generated colors. | __ |
size | string | Size of the avatar | __ |
customSize | string | customSize of the avatar, example -> customSize="62px" | __ |
rounded | AvatarRounded | Set border radius | "full" |
onClick | AvatarOnclick | Mouse click event | __ |
className | string | Name of the CSS class you want to add to this component alongside the default sb-avatar. | __ |
Avatar Colors
type AvatarColors = "primary" | "secondary" | "danger" | "info" | "success" | "warning";
Avatar Rounded
type AvatarRounded = "sm" | "md" | "lg" | "full" | "none";
Avatar onClick
type AvatarOnclick = (e: SyntheticEvent<any, Event>) => any;